raise IndexError("Index out of range.")
测试:
>>> a = Point(1,2)
>>> a[0], a[1]
(1, 2)
>>> a[-1], a[-2]
(2, 1)
>>> a[0] = 5
>>> a
Point(5, 2)
>>> a[1] = 3
>>> a
Point(5, 3)
>>> [i for i in a]
[5, 3]
>>> x, y = a
>>> x
5
>>> y
3
>>> b = iter(a)
>>> next(b)
5
>>> next(b)
3
>>> next(b)
Traceback (most recent call last):
File "<pyshell#67>", line 1, in <module>
next(b)
StopIteration
#### \_\_iter\_\_
#### \_\_next\_\_
共同定义一个对象的迭代行为,迭代器必须实现\_\_iter\_\_()方法,该方法返回迭代器自身,或者返回一个新的迭代器对象。\_\_next\_\_()方法返回迭代器的下一个元素。
class Point:
def init(self, x=0, y=0):
self.x, self.y = x, y
self.index = 0
def repr(self):
return f’Point({self.x}, {self.y})’
def iter(self):
self.index = 0
return self
def next(self):
if self.index < 2:
result = self.y if self.index else self.x
self.index += 1
return result
else:
raise StopIteration
测试:
>>> a = Point(5, 3)
>>> x, y = a
>>> x, y
(5, 3)
>>> next(a)
Traceback (most recent call last):
File "<pyshell#115>", line 1, in <module>
next(a)
File "<pyshell#111>", line 16, in \_\_next\_\_
raise StopIteration
StopIteration
>>> a = Point(5, 3)
>>> next(a)
5
>>> next(a)
3
>>> a
Point(5, 3)
>>> a.x
5
>>> next(a)
Traceback (most recent call last):
File "<pyshell#121>", line 1, in <module>
next(a)
File "<pyshell#111>", line 16, in \_\_next\_\_
raise StopIteration
StopIteration
>>> a[0]
Traceback (most recent call last):
File "<pyshell#122>", line 1, in <module>
a[0]
TypeError: 'Point' object is not subscriptable
对于点类说,可迭代魔法方法完全可弃用;因为使用\_\_getitem\_\_方法和iter()函数已有此功能。
#### \_\_len\_\_
求长度的方法,原义就是计算可迭代对象元素的个数;点类的长度就是2。
def __len__(self):
return 2
#### \_\_neg\_\_
求相反数的方法,也就是单目的**“ - ”**符号;重载为横纵坐标都取相反数。
def __neg__(self):
return Point(-self.x, -self.y)
#### \_\_pos\_\_
这是单目的**“ + ”**符号,一般无需重新定义;但是我们还是把它重载成穿过点的横纵两条直线上所有的整数点坐标,还是有点象形的,如一个十字架。
class Point:
def init(self, x=0, y=0):
self.x, self.y = x, y
def repr(self):
return f’Point({self.x}, {self.y})’
def pos(self):
n = 0
while True:
yield Point(n, self.y), Point(self.x, n)
n += 1
测试:
>>> a = Point(2, 4)
>>> b = +a
>>> next(b)
(Point(0, 4), Point(2, 0))
>>> next(b)
(Point(1, 4), Point(2, 1))
>>> next(b)
(Point(2, 4), Point(2, 2))
>>> next(b)
(Point(3, 4), Point(2, 3))
>>> next(b)
(Point(4, 4), Point(2, 4))
>>> next(b)
(Point(5, 4), Point(2, 5))
>>> b = +a
>>> horizontal = [next(b)[0] for \_ in range(5)]
>>> horizontal
[Point(0, 4), Point(1, 4), Point(2, 4), Point(3, 4), Point(4, 4)]
>>> b = +a
>>> vertical = [next(b)[1] for \_ in range(5)]
>>> vertical
[Point(2, 0), Point(2, 1), Point(2, 2), Point(2, 3), Point(2, 4)]
这种设计返回的点太多,使用并不方便。有必要改成只返回上下左右相邻的四个点:
class Point:
def init(self, x=0, y=0):
self.x, self.y = x, y
def repr(self):
return f’Point({self.x}, {self.y})’
def pos(self):
return Point(self.x, self.y+1), Point(self.x, self.y-1), Point(self.x-1, self.y), Point(self.x+1, self.y)
#### \_\_abs\_\_
求绝对值的方法,重载时定义为把横纵坐标都取绝对。
def __abs__(self):
return Point(*map(abs,(self.x, self.y)))
以上三种方法不改变类自身,注意以下写法会使类改变自身:
def __neg__(self):
self.x = -self.x
return self
def __pos__(self):
self.y = -self.y
return self
def __abs__(self):
self.x, self.y = map(abs,(self.x, self.y))
return self
#### \_\_bool\_\_
布尔值方法,重载时定义为点处在坐标系第一象限及其边界上,就返回True;否则返回False。
def __bool__(self):
return self.x>=0 and self.y>=0
#### \_\_call\_\_
这个魔术方法比较特殊,它允许一个类像函数一样被调用;我们借此定义一个点的移动。
def __call__(self, dx=0, dy=0):
return Point(self.x + dx, self.y + dy)
测试:
>>> a = Point(-5,5)
>>> b = a(3, 2)
>>> b
Rc(-2, 7)
>>> b = b(3, 2)
>>> b
Rc(1, 9)
>>> a
Rc(-5, 5)
扩展一下\_\_call\_\_方法,让它除了能移动点还能计算点到点的实际距离:
def __call__(self, dx=0, dy=0, distance=False):
if distance:
return ((self.x-dx)**2 + (self.y-dy)**2)**0.5
return Point(self.x + dx, self.y + dy)
测试:
>>> a = Point(3,4)
>>> a(0,0,True)
5.0
>>> len(a)
5
>>> a(\*a(1, 1), True)
1.4142135623730951
>>> a
Rc(3, 4)
>>> a(2, 3, True)
1.4142135623730951
注:一旦定义了\_\_call\_\_这个方法,\_\_pos\_\_方法就能改进得更简洁。
class Point:
def init(self, r=0, c=0):
self.x, self.y = r, c
def repr(self):
return f’Point({self.x}, {self.y})’
def pos(self):
return self(0, 1), self(0, -1), self(-1), self(1)
def call(self, dx=0, dy=0):
return Point(self.x + dx, self.y + dy)
测试:
>>> a=Point()
>>> +a
(Point(0, 1), Point(0, -1), Point(-1, 0), Point(1, 0))
>>> +Point() # 直接返回上下左右四个方向
(Point(0, 1), Point(0, -1), Point(-1, 0), Point(1, 0))
>>> b = Point(7, 8)
>>> +b
(Point(7, 9), Point(7, 7), Point(6, 8), Point(8, 8))
最后,综合以上所有**有用的**魔术方法,代码如下:
class Point:
def init(self, x=0, y=0):
self.x, self.y = x, y
def repr(self):
return f’Point({self.x}, {self.y})’
def str(self):
return f’({self.x}, {self.y})’
def getitem(self, index):
if index in range(-2,2):
return self.y if index in (1,-1) else self.x
raise IndexError(“Index out of range”)
def setitem(self, index, value):
if index in (0, -2):
self.x = value
elif index in (1, -1):
self.y = value
else:
raise IndexError(“Index out of range.”)
def len(self):
return 2
def abs(self):
return Point(*map(abs,(self.x, self.y)))
def bool(self):
return self.x>=0 and self.y>=0
def neg(self):
return Point(-self.x, -self.y)
def pos(self):
return self(0, 1), self(0, -1), self(-1), self(1)
def call(self, dx=0, dy=0):
return Point(self.x + dx, self.y + dy)
### 重载运算符
python中,常用的运算符都有对应的魔法方法可以重新定义新的运算操作。
#### 比较运算符
##### 相等 ==
两个点相等,就是它俩的横纵坐标分别相等。
def __eq__(self, other):
return self.x == other.x and self.y == other.y
为使得类更强健,可以对参数other作一类型判断:
def \_\_eq\_\_(self, other):
assert(isinstance(other, Point))
return self.x == other.x and self.y == other.y
或者:
def \_\_eq\_\_(self, other):
if isinstance(other, Point):
return self.x == other.x and self.y == other.y
else:
raise TypeError("Operand must be an instance of Point")
##### 不等 !=
def __ne__(self, other):
return self.x != other.x or self.y != other.y
也可以这样表示:
>
> def \_\_ne\_\_(self, other):
> return not self.\_\_eq\_\_(other)
>
>
>
因为 not self.x == other.x and self.y == other.y 即 not self.x == other.x or not self.y == other.y 。
经测试,有了\_\_eq\_\_,\_\_ne\_\_可有可无,直接可以用 != 运算。
>>> class Point:
... def \_\_init\_\_(self, x=0, y=0):
... self.x, self.y = x, y
... def \_\_eq\_\_(self, other):
... return self.x == other.x and self.y == other.y
...
...
>>> a = Point(2, 5)
>>> b = Point(2, 5)
>>> c = Point(1, 3)
>>> a == a
True
>>> a == b
True
>>> a == c
False
>>> a != b
False
>>> b != c
True
>>> class Point:
... def \_\_init\_\_(self, x=0, y=0):
... self.x, self.y = x, y
... def \_\_eq\_\_(self, other):
... return self.x == other.x and self.y == other.y
... def \_\_ne\_\_(self, other):
... return self.x != other.x or self.y != other.y
...
...
>>> a = Point(2, 5)
>>> b = Point(2, 3)
>>> a != b
True
##### 大于和小于 >、<
坐标比大小没什么物理意义,就搞点“花样”出来:小于 < 判断左边的横坐标是否相等;大于 > 判断右边的纵坐标是否相等;但纵横坐标不能同时相等。实际用处就是判断两点是否在同一水平线或同一垂直线上。
def __lt__(self, other):
return self.x == other.x and self.y != other.y
def __gt__(self, other):
return self.x != other.x and self.y == other.y
##### 大于等于和小于等于 >=、<=
在大于小于的基础上,大于等于和小于等于就重载成计算同一水平线或垂直线上的两点的距离。即小于等于 <= 横坐标相等时计算纵坐标的差;而大于等于 >= 纵坐标相等时计算横坐标的差。
def __le__(self, other):
return self.x == other.x and self.y - other.y
def __ge__(self, other):
return self.y == other.y and self.x - other.x
测试:
>>> a = Point(2, 5)
>>> b = Point(2, 3)
>>> a <= b
2
>>> b <= a
-2
>>> a >= b
False
>>> b >= a
False
>>> a = Point(5, 1)
>>> b = Point(2, 1)
>>> a >= b
3
>>> b >= a
-3
>>> a <= b
False
b <= a
False
a < b
False
b > a
True
b < a
False
a > b
True
c = Point(2, 2)
c >= c
0
c > c
False
0 is False
False
0 == False
True
基于以上结果,只要注意对相同两点判断时,使用==False可能误判,因为0 is False,但用 is 来判断就能区别开来,is False 和 is 0 效果是不相同。所以我们把>=和<=的功能让给>和<,大于等于和小于等于重新定义为**两点的横坐标或纵坐标是否(整数)相邻**,并且为了好记忆,让 < 和 <= 管左边的横坐标,让 > 和 >= 管右边的纵坐标。修改后的所有比较运算符的重载代码如下:
class Point:
def init(self, x=0, y=0):
self.x, self.y = x, y
def repr(self):
return f’Point({self.x}, {self.y})’
def str(self):
return f’({self.x}, {self.y})’
def eq(self, other):
return self.x == other.x and self.y == other.y
def ne(self, other):
return self.x != other.x or self.y != other.y
def gt(self, other):
return self.x == other.x and self.y - other.y
def lt(self, other):
return self.y == other.y and self.x - other.x
def ge(self, other):
return self.x == other.x and abs(self.y - other.y)==1
def le(self, other):
return self.y == other.y and abs(self.x - other.x)==1
#### 位运算符
位运算符是一类专门用于处理整数类型数据的二进制位操作的运算符。
##### 位与 &
原义是对两个数的二进制表示进行按位与操作,只有当两个位都为1时,结果位才为1,否则为0。
##### 位或 |
原义是对两个数的二进制表示进行按位或操作,只要有一个位为1,结果位就为1。
== 和 != 分别表示横纵坐标 **“x,y都相等”** 和 **“至少有一个不等”**,互为反运算;
那就把 **与** & 和 **或**| 重载成 **“x,y都不相等”** 和 **“至少有一个不等”**,正好也互为反运算。
def and(self, other):
return self.x != other.x and self.y != other.y
def or(self, other):
return self.x == other.x or self.y == other.y
可以理解为:==是严格的**相等**,“与” 是严格的**不等**;“或”是不严格的**相等**,!=是不严格的**不等**。
##### 位异或 ^
原义是对两个数的二进制表示进行按位异或操作,当两个位不相同时,结果位为1;相同时为0。
那就把 **异或** ^ 重载成横纵坐标 **“x,y有且只有一个值相等”**,非常接近异或的逻辑意义。
def xor(self, other):
return self.x == other.x and self.y != other.y or self.x != other.x and self.y == other.y
##### 位取反 ~
原义是将整数的二进制每一位进行取反操作,即将0变为1,将1变为0。对一个十进制整数n来说, ~n == -n-1;有个妙用列表的索引从0开始,索引下标0,1,2,3表示列表的前4个,而~0,~1,~2,~3正好索引列表的倒数4个元素,因为它们分别等于-1, -2, -3, -4。
**取反**重载时,我们把它定义成交换坐标点的纵横坐标。
def __invert__(self):
return Point(self.y, self.x)
测试:
>>> a = Point(1, 5)
>>> a
Point(1, 5)
>>> ~a
Point(5, 1)
>>> a
Point(1, 5)
>>> a = ~a
>>> a
Point(5, 1)
##### 左位移 <<
位移运算符的原义是将整数的二进制位全部左移或右移指定的位数。左移时,低位溢出的位被丢弃,高位空出的位用0填充;左移运算相当于对数值进行乘以2的运算 。
##### 右位移 >>
右移运算对于有符号整数,右移时会保留符号位(即最高位),并在左侧填充与符号位相同的位。对于无符号整数,右移时左侧填充0;每次右移相当于将数值整除2的运算。
**位移**运算符重载时采用和比较运算符重载时相同的箭头指向性,即左位移管横坐标的移动,右位移管纵坐标的位移,此时other为整数,正整数指坐标点向右移动或向上移动;负整数刚好相反。
def __lshift__(self, other):
return Point(self.x + other, self.y)
def __rshift__(self, other):
return Point(self.x, self.y + other)
测试:
>>> a = Point(5, 1)
a
Point(5, 1)
a >> 4
Point(5, 5)
a << -4
Point(1, 1)
a
Point(5, 1)
a >>= 4
a
Point(5, 5)
a <<= -4
a
Point(1, 5)
>>> a = Point(1, 5)
>>> b = Point(1, 1)
>>> a > b
4
>>> if (n:=a>b):
... a >>= -n
...
...
>>> a == b
True
**位移运算重载后,同时位移并赋值功能也生效,即 >>= 和 <<= 也同时被重载。** 这种赋值功能也可以用 \_\_ilshift\_\_(self, other),\_\_irshift\_\_(self, other);大部分方法都有字母 **i** 开头对应的运算并赋值的方法,如\_\_iadd\_\_、\_\_isub\_\_、\_\_imul\_\_、\_\_ior\_\_......等等。
与\_\_call\_\_方法调用的比较:\_\_call\_\_更灵活可以同时改变横、纵坐标。
>>> a = Point(-5,5)
>>> a << 5
Rc(0, 5)
>>> a
Rc(-5, 5)
>>> a(5)
Rc(0, 5)
>>> a
Rc(-5, 5)
>>> a(0,-5)
Rc(-5, 0)
>>> a >> -5
Rc(-5, 0)
>>> a
Rc(-5, 5)
综合所有位运算符,代码如下:
class Point:
def init(self, x=0, y=0):
self.x, self.y = x, y
def repr(self):
return f’Point({self.x}, {self.y})’
def and(self, other):
return self.x != other.x and self.y != other.y
def or(self, other):
return self.x == other.x or self.y == other.y
def xor(self, other):
return self.x == other.x and self.y != other.y or self.x != other.x and self.y == other.y
def invert(self):
return Point(self.y, self.x)
def lshift(self, other):
return Point(self.x + other, self.y)
def rshift(self, other):
return Point(self.x, self.y + other)
#### 算术运算符
算术运算很简单,除了加减乘除+,-,\*,/,还有幂运算 \*\*、取模 %、整除 // 等。
##### 加 +
**加法**很明显重载成坐标值的相加最接近加法的本义:
def \_\_add\_\_(self, other):
return Point(self.x + other.x, self.y + other.y)
我们可以让other的定义域进一步扩大不仅限于是个点类,只要符合指定条件都可以“相加”,即移动为另一个点;如果“点”和不符合条件的对象相加,则返回 None。
指定条件为 **hasattr**(other, **'\_\_getitem\_\_'**) **and** **len**(other)==2 ,重载定义如下:
class Point:
def init(self, x=0, y=0):
self.x, self.y = x, y
def repr(self):
return f’Point({self.x}, {self.y})’
def getitem(self, index):
if index in range(-2,2):
return self.y if index in (1,-1) else self.x
raise IndexError(“Index out of range”)
def len(self):
return 2
def call(self, dx=0, dy=0):
return Point(self.x + dx, self.y + dy)
def add(self, other):
if hasattr(other, ‘getitem’) and len(other)==2:
return self.call(*map(int, other))
测试:
>>> a = Point(3,4)
>>> b = Point(2,-2)
>>> a + b
Point(5, 2)
>>> a + (1,1)
Point(4, 5)
>>> a + '11'
Point(4, 5)
>>> a + '1' # None
与调用\_\_call\_\_方法的比较:
>>> p = Point(5, 5)
>>> d = Point(2, 3)
>>> p + d
Point(7, 8)
>>> p(d.x, d.y)
Point(7, 8)
>>> p(2, 3)
Point(7, 8)
>>> p
Point(5, 5)
##### 减 -
因为减一个数就是加它的相反数,所以没必要把减法运算重载成和加法一样的模式;我们可以把**减法**重载成两点之间的距离,重载定义为:
class Point:
def init(self, x=0, y=0):
self.x, self.y = x, y
def repr(self):
return f’Point({self.x}, {self.y})’
def getitem(self, index):
if index in range(-2,2):
return self.y if index in (1,-1) else self.x
raise IndexError(“Index out of range”)
def len(self):
return 2
def sub(self, other):
if hasattr(other, ‘getitem’) and len(other)==2:
dx, dy = tuple(map(float, other))
return ((self.x-dx)**2 + (self.y-dy)**2)**0.5
测试:
>>> a = Point(3,4)
>>> a - Point()
5.0
>>> a - (4,5)
1.4142135623730951
>>> a - '44'
1.0
>>> a - 3 # None
##### 乘 \*
**乘法**就重载为判断两点是否整数相邻,即: self >= other or self <= other
class Point:
def init(self, x=0, y=0):
self.x, self.y = x, y
def repr(self):
return f’Point({self.x}, {self.y})’
def ge(self, other):
return self.x == other.x and abs(self.y - other.y)==1
def le(self, other):
return self.y == other.y and abs(self.x - other.x)==1
def call(self, dx=0, dy=0):
return Point(self.x + dx, self.y + dy)
def mul(self, other):
return self >= other or self <= other
测试:
>>> P0 = Point(3, 5)
>>> lst = (0,1), (0,-1), (-1,0), (1,0), (1,1)
>>> P5 = [P0(x,y) for x,y in lst]
>>> P5
[Point(3, 6), Point(3, 4), Point(2, 5), Point(4, 5), Point(4, 6)]
>>> [P0\*p for p in P5]
[True, True, True, True, False]
##### 除 /
**除法**就重载为判断在同一水平线或垂直线上的两点,是正序还是反序;正序是指前左后右或前下后上,返回1;反序则相反,前右后左或前上后下,返回-1;不符条件的,则返回False。
class Point:
def init(self, x=0, y=0):
self.x, self.y = x, y
def repr(self):
return f’Point({self.x}, {self.y})’
def gt(self, other):
return self.x == other.x and self.y - other.y
def lt(self, other):
return self.y == other.y and self.x - other.x
def xor(self, other):
return self.x == other.x and self.y != other.y or self.x != other.x and self.y == other.y
def truediv(self, other):
return (self^other) and (1 if (self<other)<0 or (self>other)<0 else -1)
测试:
>>> a = Point(1, 2)
>>> b = Point(5, 2)
>>> c = Point(5, 5)
>>> a / b , b / a, b / c, c / b
(1, -1, 1, -1)
>>> a / c, c / a, a / a, c / c
(False, False, False, False)
##### 幂 \*\*
**幂**运算就重载为返回在同一水平线或垂直线上的两点之间的点;不符合条件的,则返回None。
class Point:
def init(self, x=0, y=0):
self.x, self.y = x, y
def repr(self):
return f’Point({self.x}, {self.y})’
def gt(self, other):
return self.x == other.x and self.y - other.y
def lt(self, other):
return self.y == other.y and self.x - other.x
def xor(self, other):
return self.x == other.x and self.y != other.y or self.x != other.x and self.y == other.y
def truediv(self, other):
return (self^other) and (1 if (self<other)<0 or (self>other)<0 else -1)
def pow(self, other):
if self^other:
if self<other: return [Point(,self.y) for _ in range(self.x+(self/other),other.x,self/other)]
if self>other: return [Point(self.x,) for _ in range(self.y+(self/other),other.y,self/other)]
测试:
>>> a = Point(1, 2)
>>> b = Point(5, 2)
>>> c = Point(5, 5)
>>> a \*\* b
[Point(2, 2), Point(3, 2), Point(4, 2)]
>>> b \*\* a
[Point(4, 2), Point(3, 2), Point(2, 2)]
>>> b \*\* c
[Point(5, 3), Point(5, 4)]
>>> c \*\* b
[Point(5, 4), Point(5, 3)]
>>> a \*\* c # None
>>> a \*\* a # None
>>> a \*\* a == []
False
>>> a \*\* a == None
True
>>> a \*\* c == None
True
##### 取模 %
**取模**运算就重载为返回所给两点作对角线的水平矩形的另外两个端点;如果所得矩形面积为0,则返回值就是原来所给的两点。
class Point:
def init(self, x=0, y=0):
self.x, self.y = x, y
def repr(self):
return f’Point({self.x}, {self.y})’
def mod(self, other):
return [Point(self.x, dy), Point(dx, self.y)]
测试:
>>> a = Point(1, 2)
>>> b = Point(5, 5)
>>> a % b
[Point(1, 5), Point(5, 2)]
>>> (a%b)[0]
Point(1, 5)
>>> (a%b)[1]
Point(5, 2)
>>> c, d = a % b
>>> c % d
[Point(1, 2), Point(5, 5)]
>>> c % a
[Point(1, 2), Point(1, 5)]
>>> c % b
[Point(1, 5), Point(5, 5)]
示意图:
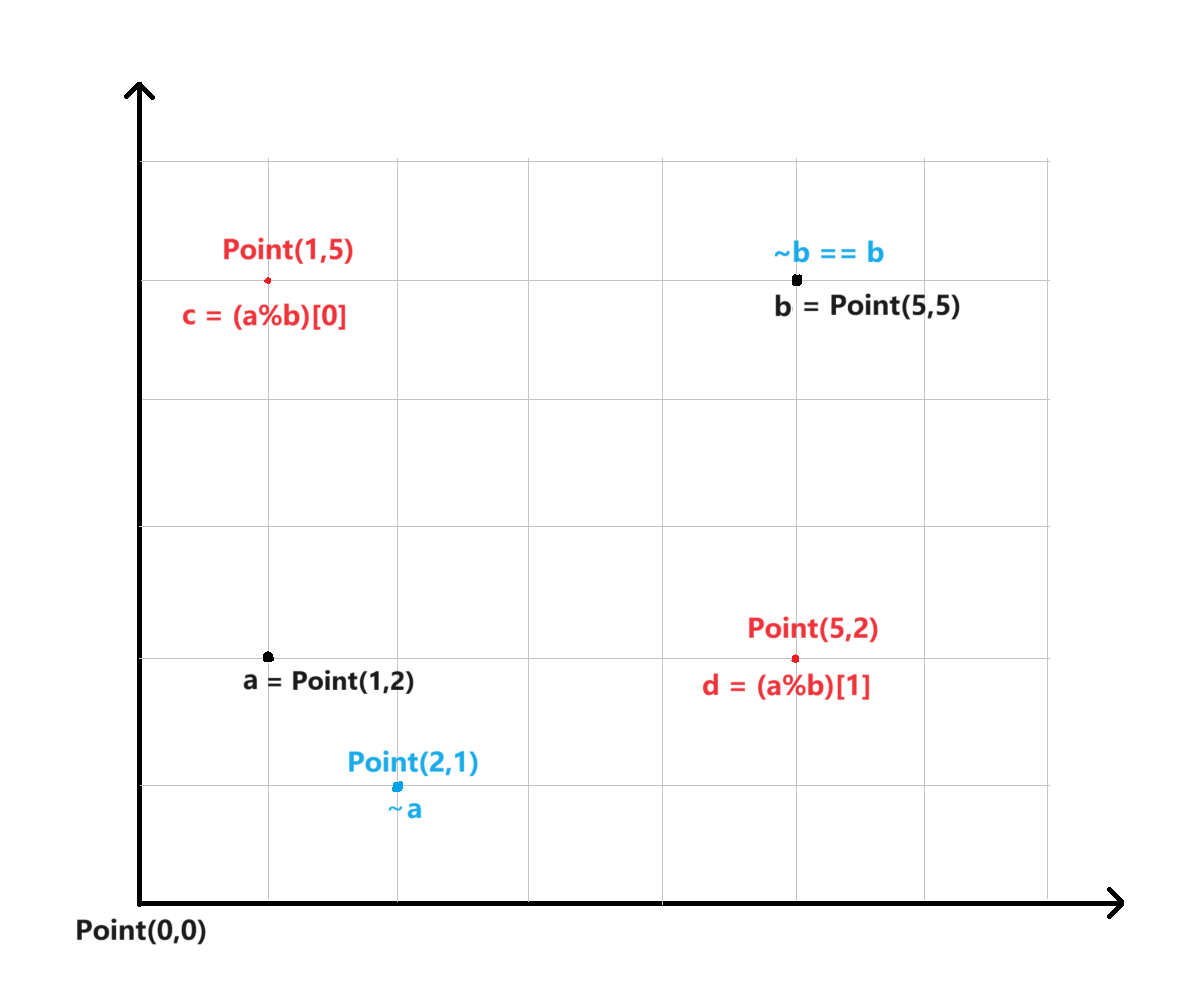
##### 整除 //
**整除**运算就重载为返回所给两点作对角线的矩形上的两组邻边上的所有点;返回点的列表也分组,如上图,一组是路径a->c->b上的点,另一级是路径a->d->b上的点。
class Point:
def init(self, x=0, y=0):
self.x, self.y = x, y
def repr(self):
return f’Point({self.x}, {self.y})’
def gt(self, other):
return self.x == other.x and self.y - other.y
def lt(self, other):
return self.y == other.y and self.x - other.x
def and(self, other):
return self.x != other.x and self.y != other.y
def xor(self, other):
return self.x == other.x and self.y != other.y or self.x != other.x and self.y == other.y
def mod(self, other):
return [Point(self.x, other.y), Point(other.x, self.y)]
def truediv(self, other):
return (self^other) and (1 if (self<other)<0 or (self>other)<0 else -1)
def pow(self, other):
if self^other:
if self<other: return [Point(,self.y) for _ in range(self.x+(self/other),other.x,self/other)]
if self>other: return [Point(self.x,) for _ in range(self.y+(self/other),other.y,self/other)]
def floordiv(self, other):
if self&other:
mod1, mod2 = self % other
return selfmod1 + [mod1] + mod1other, selfmod2 + [mod2] + mod2other
测试:
>>> a = Point(1, 2)
>>> b = Point(5, 5)
>>> a // b
([Point(1, 3), Point(1, 4), Point(1, 5), Point(2, 5), Point(3, 5), Point(4, 5)],
[Point(2, 2), Point(3, 2), Point(4, 2), Point(5, 2), Point(5, 3), Point(5, 4)])
>>> b // a
([Point(5, 4), Point(5, 3), Point(5, 2), Point(4, 2), Point(3, 2), Point(2, 2)],
[Point(4, 5), Point(3, 5), Point(2, 5), Point(1, 5), Point(1, 4), Point(1, 3)])
##### 右加 +
大部分运算符都有对应的右侧运算操作,由字母 **r** 开头,如右加\_\_radd\_\_、右减\_\_rsub\_\_、右乘\_\_rmul\_\_......等等;只有当自定义对象位于运算符号的右侧时,右运算方法才会被调用。与普通的加法相比,\_\_radd\_\_(self, other) 方法只有当 other 在加号+的左边,对象在加号+的右边才会被调用。
我们把**右加**方法重载成单目运算+差不多的规则,只是多了距离n,不一定是相邻的四点:
def __radd__(self, n):
return self(0, n), self(0, -n), self(-n), self(n)
测试:
>>> a = Point(1,2); b = Point(); n = 2
>>> a + b
Point(1, 2)
>>> b + a
Point(1, 2)
>>> a + n
Traceback (most recent call last):
File "<pyshell#29>", line 1, in <module>
a + n
AttributeError: 'int' object has no attribute 'x'
>>> n + a
(Point(1, 4), Point(1, 0), Point(-1, 2), Point(3, 2))
>>> n + b
(Point(0, 2), Point(0, -2), Point(-2, 0), Point(2, 0))
>>> 1 + b # 当n==1就返回上下左右相邻的四个点
(Point(0, 1), Point(0, -1), Point(-1, 0), Point(1, 0))
>>> 1 + b == +b
**自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。**
**深知大多数Python工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!**
**因此收集整理了一份《2024年Python开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。**
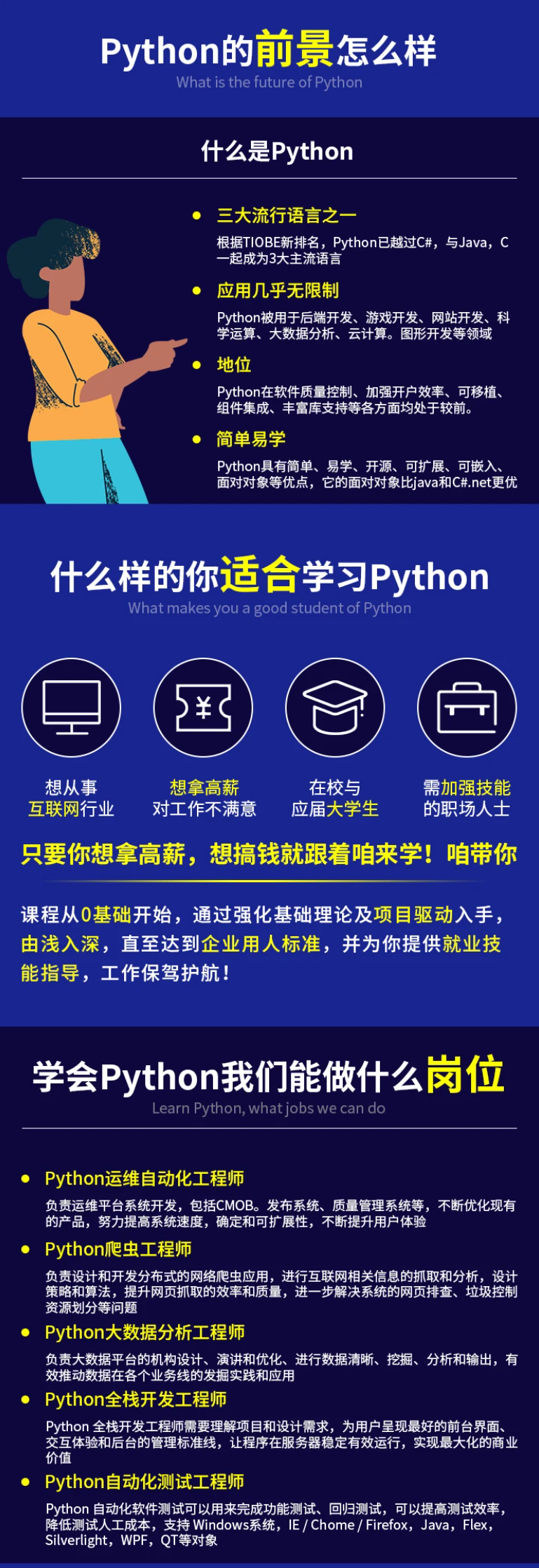
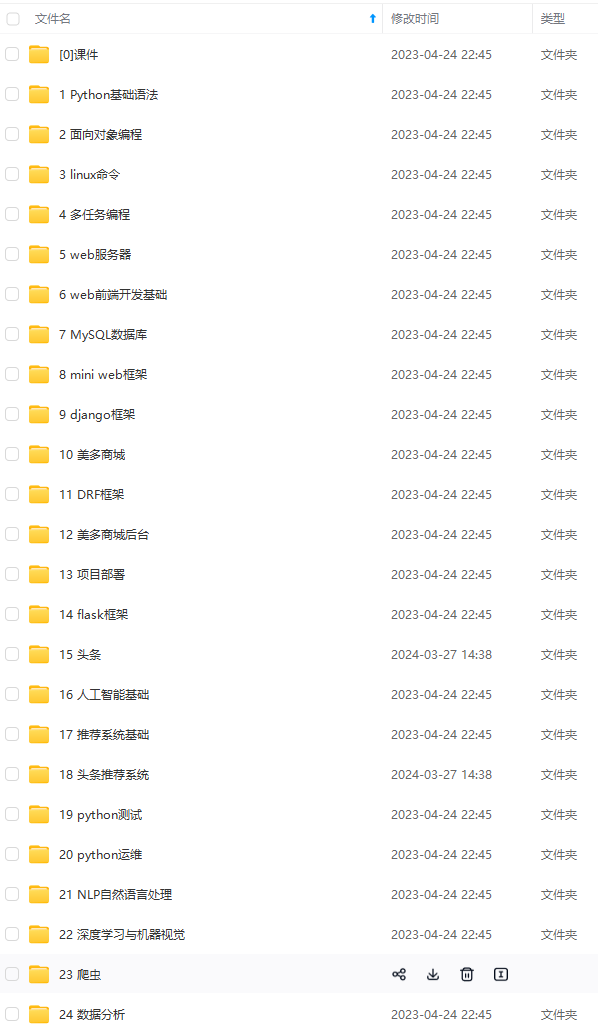
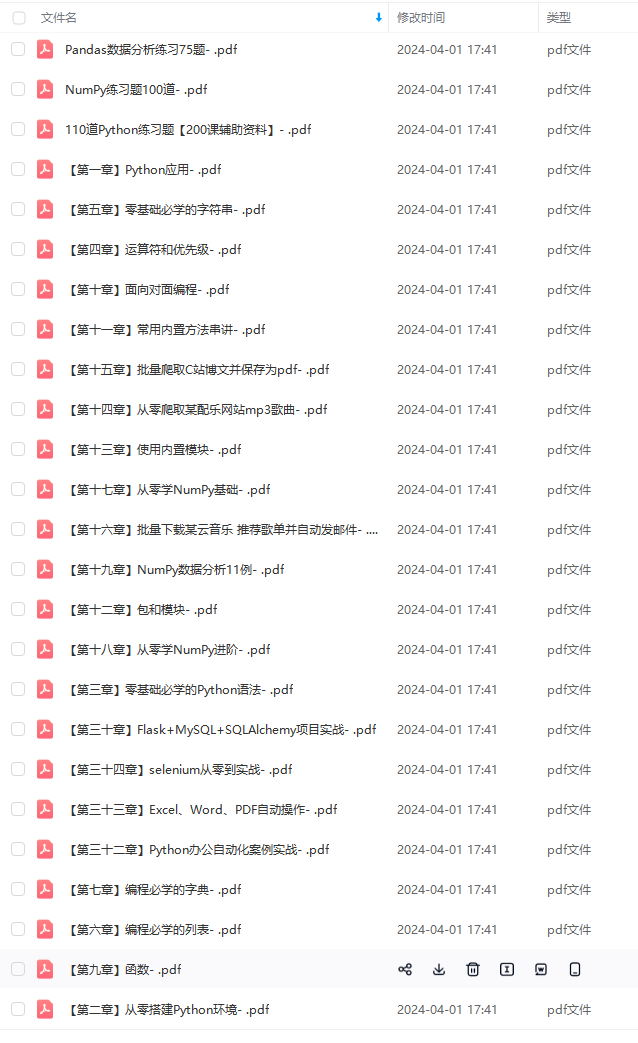
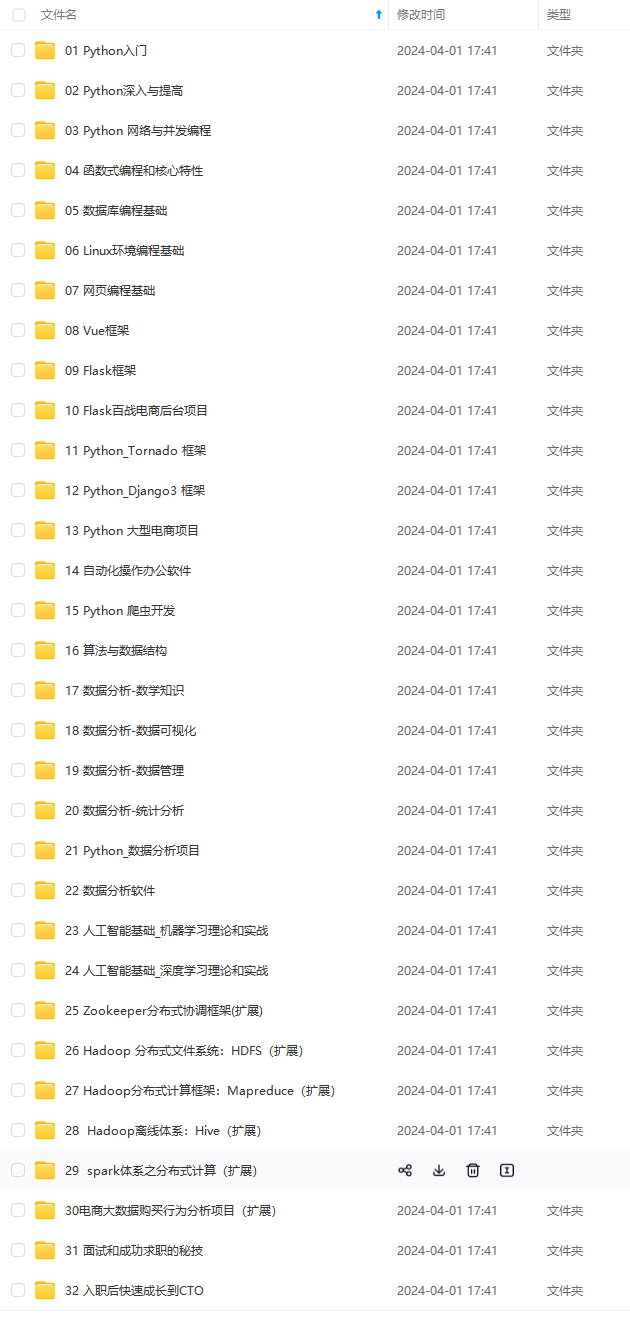
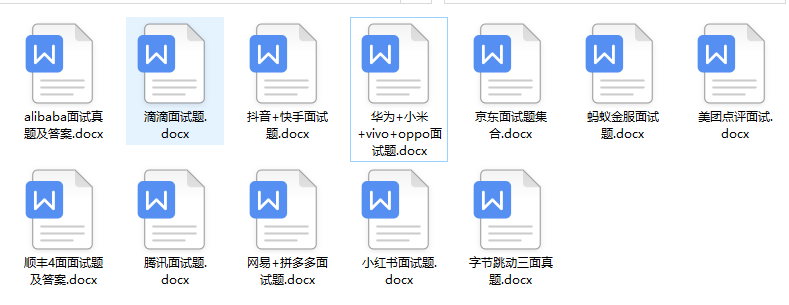
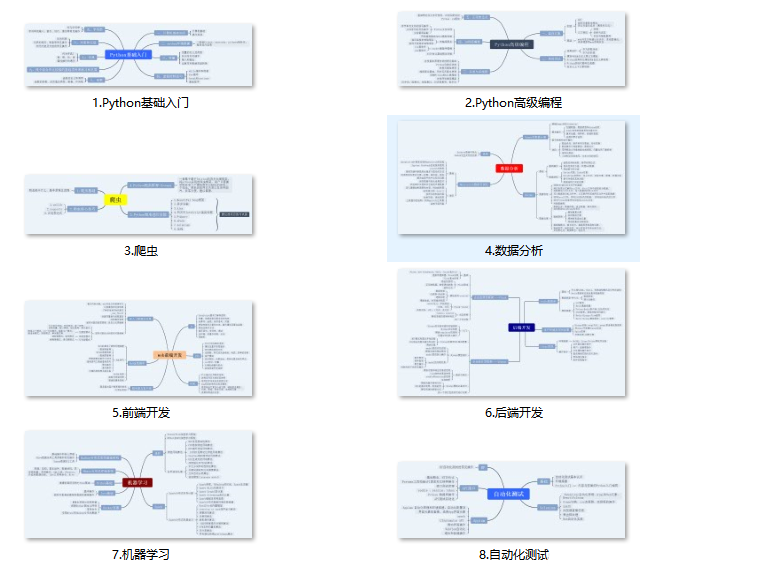
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上前端开发知识点,真正体系化!**
**由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新**
**如果你觉得这些内容对你有帮助,可以扫码获取!!!(备注:Python)**
AttributeError: 'int' object has no attribute 'x'
>>> n + a
(Point(1, 4), Point(1, 0), Point(-1, 2), Point(3, 2))
>>> n + b
(Point(0, 2), Point(0, -2), Point(-2, 0), Point(2, 0))
>>> 1 + b # 当n==1就返回上下左右相邻的四个点
(Point(0, 1), Point(0, -1), Point(-1, 0), Point(1, 0))
>>> 1 + b == +b
**自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。**
**深知大多数Python工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!**
**因此收集整理了一份《2024年Python开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。**
[外链图片转存中...(img-rk1SAvFo-1713607965794)]
[外链图片转存中...(img-BeYpMH0D-1713607965795)]
[外链图片转存中...(img-ZDYCeyty-1713607965796)]
[外链图片转存中...(img-hH8RsKtR-1713607965797)]
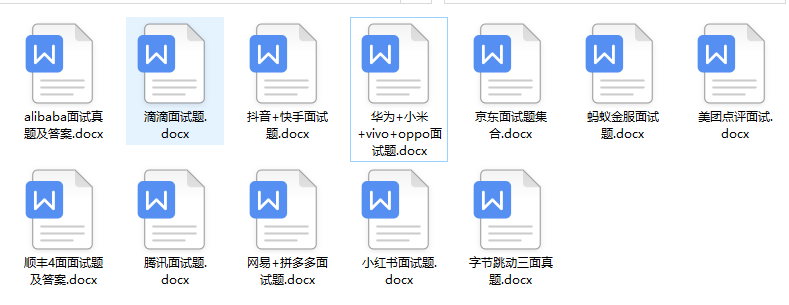
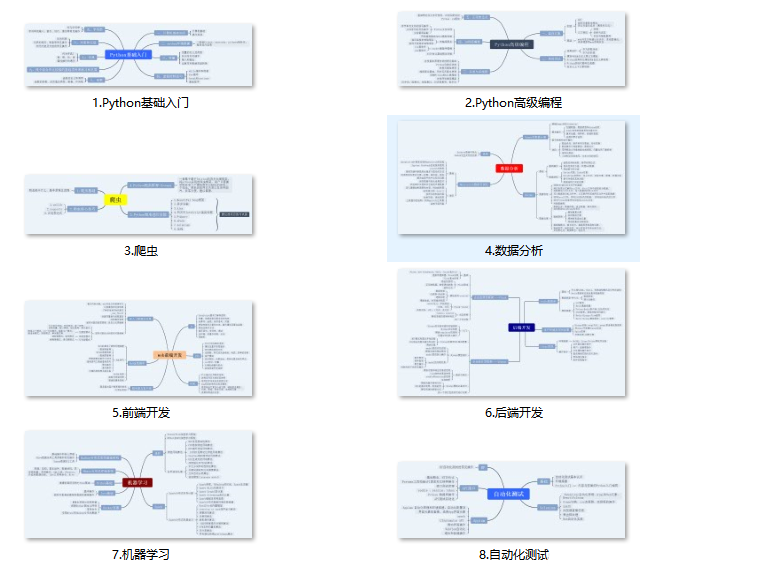
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上前端开发知识点,真正体系化!**
**由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新**
**如果你觉得这些内容对你有帮助,可以扫码获取!!!(备注:Python)**
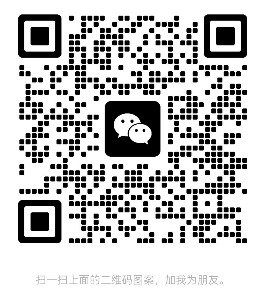
文章浏览阅读1.6k次。安装配置gi、安装数据库软件、dbca建库见下:http://blog.csdn.net/kadwf123/article/details/784299611、检查集群节点及状态:[root@rac2 ~]# olsnodes -srac1 Activerac2 Activerac3 Activerac4 Active[root@rac2 ~]_12c查看crs状态
文章浏览阅读1.3w次,点赞45次,收藏99次。我个人用的是anaconda3的一个python集成环境,自带jupyter notebook,但在我打开jupyter notebook界面后,却找不到对应的虚拟环境,原来是jupyter notebook只是通用于下载anaconda时自带的环境,其他环境要想使用必须手动下载一些库:1.首先进入到自己创建的虚拟环境(pytorch是虚拟环境的名字)activate pytorch2.在该环境下下载这个库conda install ipykernelconda install nb__jupyter没有pytorch环境
文章浏览阅读5.2k次,点赞19次,收藏28次。选择scoop纯属意外,也是无奈,因为电脑用户被锁了管理员权限,所有exe安装程序都无法安装,只可以用绿色软件,最后被我发现scoop,省去了到处下载XXX绿色版的烦恼,当然scoop里需要管理员权限的软件也跟我无缘了(譬如everything)。推荐添加dorado这个bucket镜像,里面很多中文软件,但是部分国外的软件下载地址在github,可能无法下载。以上两个是官方bucket的国内镜像,所有软件建议优先从这里下载。上面可以看到很多bucket以及软件数。如果官网登陆不了可以试一下以下方式。_scoop-cn
文章浏览阅读4.5k次,点赞2次,收藏3次。首先要有一个color-picker组件 <el-color-picker v-model="headcolor"></el-color-picker>在data里面data() { return {headcolor: ’ #278add ’ //这里可以选择一个默认的颜色} }然后在你想要改变颜色的地方用v-bind绑定就好了,例如:这里的:sty..._vue el-color-picker
文章浏览阅读640次。基于芯片日益增长的问题,所以内核开发者们引入了新的方法,就是在内核中只保留函数,而数据则不包含,由用户(应用程序员)自己把数据按照规定的格式编写,并放在约定的地方,为了不占用过多的内存,还要求数据以根精简的方式编写。boot启动时,传参给内核,告诉内核设备树文件和kernel的位置,内核启动时根据地址去找到设备树文件,再利用专用的编译器去反编译dtb文件,将dtb还原成数据结构,以供驱动的函数去调用。firmware是三星的一个固件的设备信息,因为找不到固件,所以内核启动不成功。_exynos 4412 刷机
文章浏览阅读2w次,点赞24次,收藏42次。Linux系统配置jdkLinux学习教程,Linux入门教程(超详细)_linux配置jdk
文章浏览阅读3.3k次,点赞5次,收藏19次。xlabel('\delta');ylabel('AUC');具体符号的对照表参照下图:_matlab微米怎么输入
文章浏览阅读119次。顺序读写指的是按照文件中数据的顺序进行读取或写入。对于文本文件,可以使用fgets、fputs、fscanf、fprintf等函数进行顺序读写。在C语言中,对文件的操作通常涉及文件的打开、读写以及关闭。文件的打开使用fopen函数,而关闭则使用fclose函数。在C语言中,可以使用fread和fwrite函数进行二进制读写。 Biaoge 于2024-03-09 23:51发布 阅读量:7 ️文章类型:【 C语言程序设计 】在C语言中,用于打开文件的函数是____,用于关闭文件的函数是____。
文章浏览阅读3.4k次,点赞2次,收藏13次。跟随鼠标移动的粒子以grid(SOP)为partical(SOP)的资源模板,调整后连接【Geo组合+point spirit(MAT)】,在连接【feedback组合】适当调整。影响粒子动态的节点【metaball(SOP)+force(SOP)】添加mouse in(CHOP)鼠标位置到metaball的坐标,实现鼠标影响。..._touchdesigner怎么让一个模型跟着鼠标移动
文章浏览阅读178次。项目运行环境配置:Jdk1.8 + Tomcat7.0 + Mysql + HBuilderX(Webstorm也行)+ Eclispe(IntelliJ IDEA,Eclispe,MyEclispe,Sts都支持)。项目技术:Springboot + mybatis + Maven +mysql5.7或8.0+html+css+js等等组成,B/S模式 + Maven管理等等。环境需要1.运行环境:最好是java jdk 1.8,我们在这个平台上运行的。其他版本理论上也可以。_基于java技术的停车场管理系统实现与设计
文章浏览阅读3.5k次。前言对于MediaPlayer播放器的源码分析内容相对来说比较多,会从Java-&amp;gt;Jni-&amp;gt;C/C++慢慢分析,后面会慢慢更新。另外,博客只作为自己学习记录的一种方式,对于其他的不过多的评论。MediaPlayerDemopublic class MainActivity extends AppCompatActivity implements SurfaceHolder.Cal..._android多媒体播放源码分析 时序图
文章浏览阅读2.4k次,点赞41次,收藏13次。java 数据结构与算法 ——快速排序法_快速排序法